The req object represents the HTTP request and has properties for the request query string, parameters, body, HTTP headers, and so on. In this documentation and by convention, the object is always referred to as req (and the HTTP response is res ) but its actual name is determined by the parameters to the callback function in which you’re working.
For example:
app.get('/user/:id', function(req, res) {
res.send('user ' + req.params.id);
});
But you could just as well have:
app.get('/user/:id', function(request, response) {
response.send('user ' + request.params.id);
});
-
req.baseUrl, req.body, req.cookies (req.cookies.name_of_cookie), req.hostname, req.path, etc see documentation
NOTE: if you want to see the body of the request for printing it out do the following
console.log(JSON.stringify(req.body));
if you do the following will brint out a "Object"
console.log(req.body);
For example the following handler code:
var body = JSON.stringify(req.body);
var params = JSON.stringify(req.params);
res.send("recieved your request!</br>" + "parameters: " + params + "</br>URL:" + req.url + "body: " + body);
will produce
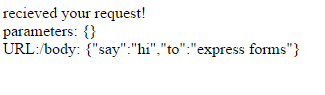
|
-
res.render, res.send, res.cookie, res.redirect, and many more
|