Facebook Login/Authentication --- how to get the social data and interactions
STEP 1 Connect your app to Facebook, initialize
window.fbAsyncInit = function() {
FB.init({
appId : '{your-app-id}',
cookie : true, // enable cookies to allow the server to access
// the session
xfbml : true, // parse social plugins on this page
version : 'v2.2' // use version 2.2
});
STEP 2 Checking the login status to see if someone's already logged into your app. During this step, you also should check to see if someone has previously logged into your app, but is not currently logged in.
FB.getLoginStatus(function(response) { statusChangeCallback(response); });
The response object that's provided to your callback contains a number of fields:
{ status: 'connected',
authResponse: {
accessToken: '...',
expiresIn:'...',
signedRequest:'...',
userID:'...' }
}
- status specifies the login status of the person using the app. The status can be one of the following:
- connected. The person is logged into Facebook, and has logged into your app.
- not_authorized. The person is logged into Facebook, but has not logged into your app.
- unknown. The person is not logged into Facebook, so you don't know if they've logged into your app.
- authResponse is included if the status is connected and is made up of the following:
- accessToken. Contains an access token for the person using the app.
- expiresIn. Indicates the UNIX time when the token expires and needs to be renewed.
- signedRequest. A signed parameter that contains information about the person using the app.
- userID is the ID of the person using the app.
// This is called with the results from from FB.getLoginStatus().
function statusChangeCallback(response) {
console.log('statusChangeCallback');
console.log(response);
// The response object is returned with a status field that lets the
// app know the current login status of the person.
// Full docs on the response object can be found in the documentation
// for FB.getLoginStatus().
if (response.status === 'connected') {
// Logged into your app and Facebook.
testAPI(); //contains some code like the one below in orange to make calls to Facebook
} else if (response.status === 'not_authorized') {
// The person is logged into Facebook, but not your app.
document.getElementById('status').innerHTML = 'Please log ' + 'into this app.';
} else {
// The person is not logged into Facebook, so we're not sure if
// they are logged into this app or not.
document.getElementById('status').innerHTML = 'Please log ' +'into Facebook.';
}
}
OPTION 1 prompt them with the Login dialog with FB.login()
FB.login(**) = function call from Facebook Javascript SDK
OPTION 2 or show them the Login Button.
<fb:login-button scope="public_profile,email" onlogin="checkLoginState();"> </fb:login-button>
This is special HTML Facebook markup that works in conjunction with having loaded Facebook Javascript SDK
AUTOMATICALLY DONE by Javascrip SDK --- NO NEW CODE HERE
At the end of the login process, an access token is generated. This access token is the thing that's passed along with every API call as proof that the call was made by a specific person from a specific app.
The Facebook SDK for JavaScript automatically handles access token storage and tracking of login status in the browser, so nothing is needed for you to store access tokens in the browser itself.
However, a common pattern is to take the access token and pass it back to a server and the server makes calls on behalf of a person. In order to get the token from the browser you can use theresponse object that's returned via FB.getLoginStatus(): -- code below is simply printing out to console ---but, you can access response.authResponse.accessToken and save in a variable that is passed back to your server web app.
FB.getLoginStatus(function(response) { if (response.status === 'connected') { console.log(response.authResponse.accessToken); } });
Now that the user is "logged in/ authenticated" you can make Facebook API calls (limited to permissions you asked for and were given). Here is some example code:
Using Javascript inside your web app (client/browser side), the easiest way to do that is with the FB.api()call. FB.api() will automatically add the access token to the call.
This code INSIDE JSP/Client side returned code:
FB.api('/me', function(response) { console.log(JSON.stringify(response)); });
Will return an array of values:
{ "id":"101540562372987329832845483", "email":"example@example.com", "first_name":"Bob", [ ... ] }
SERVER SIDE CALLS If you're making calls server side with the access token, you can use an SDK on the server to make similar calls. Many people use PHP to build web applications. You can find some examples of making server-side API calls in our PHP SDK documentation. (UNFORTUNATLEY facebook does not provide java examples---BUT SEE BELOW)
You can log people out of your app by attaching the JavaScript SDK function FB.logout to a button or a link, as follows:
FB.logout(function(response) { // Person is now logged out });
Another example directly from Facebook games authentication page-- were use
annonymous callback function for getLoginStatus
<!DOCTYPE html>
<html>
<head>
<title>Facebook Login JavaScript Example</title>
<meta charset="UTF-8">
</head>
<body>
<script>
// This is called with the results from from FB.getLoginStatus().
function statusChangeCallback(response) {
console.log('statusChangeCallback');
console.log(response);
// The response object is returned with a status field that lets the
// app know the current login status of the person.
// Full docs on the response object can be found in the documentation
// for FB.getLoginStatus().
if (response.status === 'connected')
// Logged into your app and Facebook
testAPI();
} else {
// The person is not logged into your app or we are unable to tell.
document.getElementById('status').innerHTML = 'Please log ' +
'into this app.';
}
}
// This function is called when someone finishes with the Login
// Button. See the onlogin handler attached to it in the sample
// code below.
function checkLoginState() {
FB.getLoginStatus(function(response) {
statusChangeCallback(response);
});
}
//CALL FB.init
window.fbAsyncInit = function() {
FB.init({
appId : '{your-app-id}',
cookie : true, // enable cookies to allow the server to access
// the session
xfbml : true, // parse social plugins on this page
version : 'v2.8' // use graph api version 2.8
});
// NOW that we've initialized the JavaScript SDK, we call
// FB.getLoginStatus(). This function gets the state of the
// person visiting this page and can return one of three states to
// the callback you provide. They can be:
//
// 1. Logged into your app ('connected')
// 2. Logged into Facebook, but not your app ('not_authorized')
// 3. Not logged into Facebook and can't tell if they are logged into
// your app or not.
//
// These three cases are handled in the callback function.
FB.getLoginStatus(function(response) {
statusChangeCallback(response);
});
};
// Load the SDK asynchronously
(function(d, s, id) {
var js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) return;
js = d.createElement(s); js.id = id;
js.src = "https://connect.facebook.net/en_US/sdk.js";
fjs.parentNode.insertBefore(js, fjs);
}(document, 'script', 'facebook-jssdk'));
// Here we run a very simple test of the Graph API after login is
// successful. See statusChangeCallback() for when this call is made.
function testAPI() {
console.log('Welcome! Fetching your information.... ');
FB.api('/me', function(response) {
console.log('Successful login for: ' + response.name);
document.getElementById('status').innerHTML =
'Thanks for logging in, ' + response.name + '!';
});
}
</script>
<!-- Below we include the Login Button social plugin. This button uses
the JavaScript SDK to present a graphical Login button that triggers
the FB.login() function when clicked. -->
<fb:login-button scope="public_profile,email" onlogin="checkLoginState();">
</fb:login-button>
<div id="status"> </div>
</body>
</html>
|
NOTE: WE ARE NOT going to do the following but, wanted you to know this is possible
In order to create a personalized user experience, Facebook sends your app information about the user. This information is passed to your Canvas URL using HTTP POST within a single signed_request parameter which contains a base64url encoded JSON object.
When a user first accesses your app, the signed_request parameter contains a limited amount of user data:
Name |
Description |
user |
A JSON array containing the locale string, country string and the age object (containing the min and max numbers of the age range) for the current user. |
algorithm |
A JSON string containing the mechanism used to sign the request. |
issued_at |
A JSON number containing the Unix timestamp when the request was signed. |
In order to gain access to all the user information available to your app by default (like the user's Facebook ID), the user must authorize your app. We recommend that you use the OAuth Dialog for Apps on Facebook.com. You invoke this dialog by redirecting the user's browser to the following URL (replacing the YOUR_APP_ID and YOUR_CANVAS_PAGE with the correct values found in the Developer App):
THIS IS FOR ONLY basic information
https://www.facebook.com/dialog/oauth?client_id=YOUR_APP_ID&redirect_uri=YOUR_CANVAS_PAGE
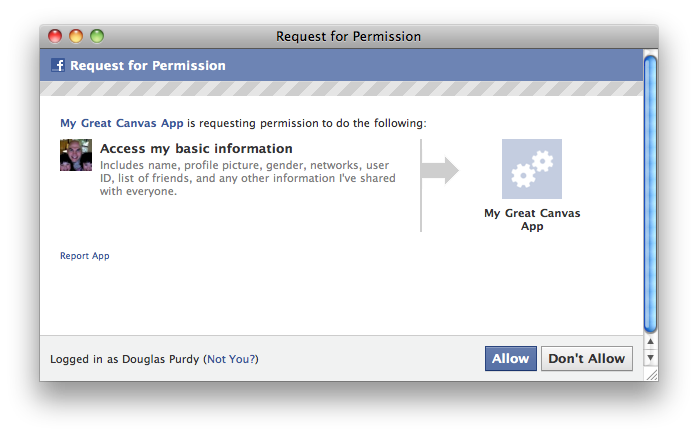
By default, the user is asked to authorize the app to access basic information that is available publicly or by default on Facebook. If your app needs more than this basic information (but, this leads to using the graph api to get a lot of social data) to function, you must request specific permissions from the user (i.e. email and news feed is not part of the basic information permission). This is accomplished by adding a scope parameter to the OAuth Dialog request followed by comma separated list of the required permissions. The following example shows how to ask for access to user's email address and their news feed:
THIS IS FOR EMAIL + NEWS FEED
https://www.facebook.com/dialog/oauth?client_id=YOUR_APP_ID&redirect_uri=YOUR_CANVAS_PAGE&scope=email,read_stream
NOTE: The signed_request parameter is utilized to share information between Facebook and app in a number of different scenarios:
- A signed_request is passed to Apps on Facebook.com when they are loaded into the Facebook environment
- A signed_request is passed to any app that has registered an Deauthorized Callback in the Developer App whenever a given user removes the app using the App Dashboard
- A signed_request is passed to apps that use the Registration Plugin whenever a user successfully registers with their app
The signed_request parameter is the concatenation of a HMAC SHA-256 signature string, a period (.), and a base64url encoded JSON object. It looks something like this (without the newlines):
vlXgu64BQGFSQrY0ZcJBZASMvYvTHu9GQ0YM9rjPSso
.
eyJhbGdvcml0aG0iOiJITUFDLVNIQTI1NiIsIjAiOiJwYXlsb2FkIn0
NOTE: Because of the way that we currently load iframes for Apps on Facebook.com, it is important that you navigate the top window of the user's browser to the OAuth Dialog. Many apps do this by sending a script fragment to the user's browser setting the top.location.href property to the dialog URL.
Next Step --how to parse the signed_request parameter --OAuth
Please see the PHP example
Because your application is being loaded in an iframe, returning a 302 to redirect the user to the OAuth Dialog will be unsuccessful. Instead you must redirect by setting the Javascript window.top.location property, which causes the parent window to redirect to the OAuth Dialog URL:
<script>
var oauth_url = 'https://www.facebook.com/dialog/oauth/';
oauth_url += '?client_id=YOUR_APP_ID';
oauth_url += '&redirect_uri=' + encodeURIComponent('https://apps.facebook.com/YOUR_APP_NAMESPACE/');
oauth_url += '&scope=COMMA_SEPARATED_LIST_OF_PERMISSION_NAMES';
window.top.location = oauth_url;
</script>
Note that the OAuth Dialog URL doesn't have to be generated on the client side, it is done so hear for clarity. You may generate this URL on the server-side, but it must be passed to the Javascript property window.top.location to affect the redirection.
|