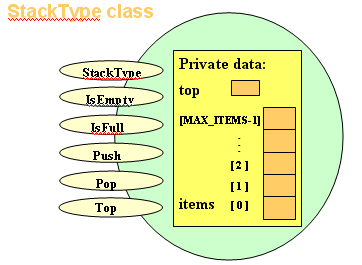
// Class specification for Stack ADT in file StackType.h
// uses Array of specified maximum size to store items
class FullStack // Exception class thrown by
// Push when stack is full
{};
class EmptyStack // Exception class thrown by
// Pop and Top when stack is empty
{};
#include "ItemType.h"
class StackType
{
public:
StackType( );
// Class constructor.
bool IsFull () const;
// Function: Determines whether the stack is full.
// Pre: Stack has been initialized
// Post: Function value = (stack is full)
bool IsEmpty() const;
// Function: Determines whether the stack is empty.
// Pre: Stack has been initialized.
// Post: Function value = (stack is empty)
void Push( ItemType item );
// Function: Adds newItem to the top of the stack.
// Pre: Stack has been initialized.
// Post: If (stack is full), FullStack exception is thrown;
// otherwise, newItem is at the top of the stack.
void Pop();
// Function: Removes top item from the stack.
// Pre: Stack has been initialized.
// Post: If (stack is empty), EmptyStack exception is thrown;
// otherwise, top element has been removed from stack.
ItemType Top();
// Function: Returns a copy of top item on the stack.
// Pre: Stack has been initialized.
// Post: If (stack is empty), EmptyStack exception is thrown;
// otherwise, top element has been removed from stack.
private:
int top;
ItemType items[MAX_ITEMS];
};
// File: StackType.cpp
#include "StackType.h"
#include
StackType::StackType( )
{
top = -1;
}
bool StackType::IsEmpty() const
{
return(top = = -1);
}
bool StackType::IsFull() const
{
return (top = = MAX_ITEMS-1);
}
void StackType::Push(ItemType newItem)
{
if( IsFull() )
throw FullStack():
top++;
items[top] = newItem;
}
void StackType::Pop()
{
if( IsEmpty() )
throw EmptyStack();
top--;
}
ItemType StackType::Top()
{
if (IsEmpty())
throw EmptyStack();
return items[top];
}
|