A class template allows the compiler to generate
multiple versions of a class type by using type parameters.
The formal parameter appears in the class template definition,
and the actual parameter appears in the client code. Both are enclosed
in pointed brackets, < >.
|
The actual parameter to the template
is a data type. Any type can be used, either built-in or
user-defined.
When creating class template Put .h and .cpp in same file or
Have .h include .cpp file
|
Example: Creating a class template for a stack so that can declare
to hold different data types
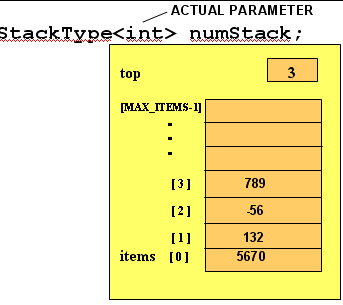
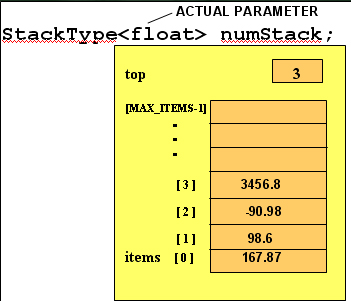
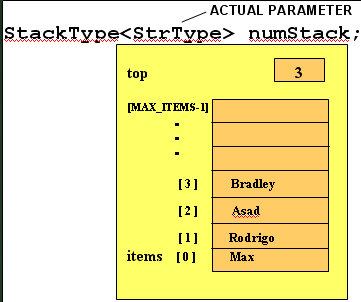
|
How to implement ....e.g. a stack
(compare to non-template
version of a stack)
//--------------------------------------------------------
// CLASS TEMPLATE DEFINITION
//--------------------------------------------------------
#include "ItemType.h" // for MAX_ITEMS and ItemTyp
template <class ItemType> // formal parameter list
class StackType
{
public:
StackType( );
bool IsEmpty( ) const;
bool IsFull( ) const;
void Push( ItemType item );
void Pop( ItemType& item );
ItemType Top( );
private:
int top;
ItemType items[MAX_ITEMS];
};
//--------------------------------------------------------
// SAMPLE CLASS MEMBER FUNCTIONS
//--------------------------------------------------------
template <class ItemType> // formal parameter list
StackType<ItemType>::StackType( )
{
top = -1;
}
template <class ItemType> // formal parameter list
void StackType<ItemType>::Push ( ItemType newItem )
{
if (IsFull())
throw FullStack();
top++;
items[top] = newItem; // STATIC ARRAY IMPLEMENTATION
}
|
Better Alternative .....dynamic array implementation (using pointers)
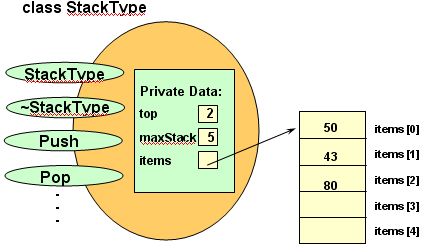
// StackType class template
template <class ItemType>
class StackType
{
public:
StackType(int max ); // max is stack size
StackType(); // Default size is 500
// Rest of the prototypes go here.
private:
int top;
int maxStack; // Maximum number of stack items
ItemType* items; // Pointer to dynamically
// allocated memory
};
// Templated StackType class variables declared
StackType<int> myStack(100);
// Stack of at most 100 integers.
StackType<float> yourStack(50);
// Stack of at most 50 floating point values.
StackType<char> aStack;
// Stack of at most 500 characters.
// Implementation of member function templates
template <class ItemType>
StackType<ItemType>::StackType(int max)
{
maxStack = max;
top = -1;
items = new ItemType[maxStack];
}
template <class ItemType>
StackType<ItemType>::StackType()
{
maxStack = 500;
top = -1;
items = new ItemType[max];
}
|