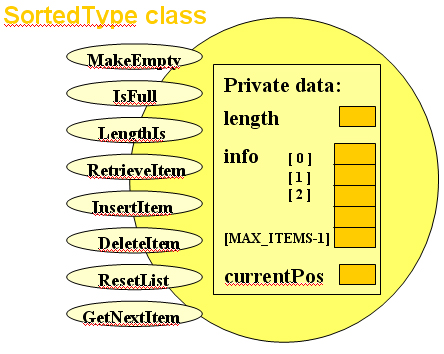 |
Which member function specifications and implementations must
change to ensure that any instance of the Sorted List ADT remains
sorted at all times?
InsertItem
DeleteItem
|
InsertItem Algoritm
- Find proper location for the new element in the sorted list.
- Create space for the new element by moving down all the list
elements that will follow it.
- Put the new element in the list.
- Increment length.
void SortedType :: InsertItem ( ItemType item )
{ bool moreToSearch ;
int location = 0 ;
// find proper location for new element
moreToSearch = ( location < length ) ;
while ( moreToSearch )
{ switch ( item.ComparedTo( info[location] ) )
{ case LESS : moreToSearch = false ;
break ;
case GREATER : location++ ;
moreToSearch = ( location < length ) ;
break ;
}
} // make room for new element in sorted list
for ( int index = length ; index > location ; index-- )
info [ index ] = info [ index - 1 ] ;
info [ location ] = item ;
length++ ;
}
|
DeleteItem Algoritm
- Find the location of the element to be deleted from the sorted
list.
- Eliminate space occupied by the item being deleted by moving
up all the list elements that follow it.
- Decrement length.
void SortedType :: DeleteItem ( ItemType item )
{
int location = 0 ;
// find location of element to be deleted
while ( item.ComparedTo ( info[location] ) != EQUAL )
location++ ;
// move up elements that follow deleted item in sorted list
for ( int index = location + 1 ; index < length; index++ )
info [ index - 1 ] = info [ index ] ;
length-- ;
}
|
Improving Retrieve Algoritm
- Recall that with the Unsorted List ADT we examined each list
element beginning with info[ 0 ], until we either found a matching
key, or we had examined all the elements in the Unsorted List.
How can the searching algorithm be improved
for Sorted List ADT?
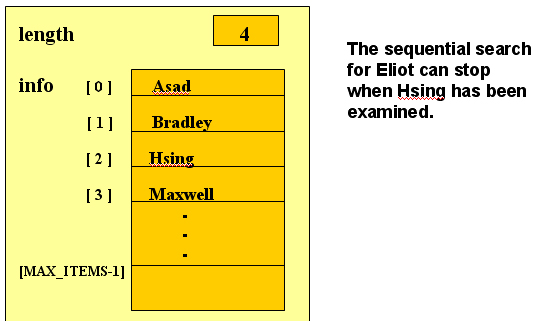
|