void SortedType::RetrieveItem ( ItemType& item, bool& found )
// Pre: Key member of item is initialized.
// Post: If found, item’s key matches an element’s key in the list
// and a copy of that element has been stored in item; otherwise,
// item is unchanged.
{ int midPoint ;
int first = 0;
int last = length - 1 ;
bool moreToSearch = ( first <= last ) ;
found = false ;
while ( moreToSearch && !found )
{ midPoint = ( first + last ) / 2 ; // INDEX OF MIDDLE ELEMENT
switch ( item.ComparedTo( info [ midPoint ] ) )
{
case LESS : . . . // LOOK IN FIRST HALF NEXT
case GREATER : . . . // LOOK IN SECOND HALF NEXT
case EQUAL : . . . // ITEM HAS BEEN FOUND
}
}
}
Binary Search Trace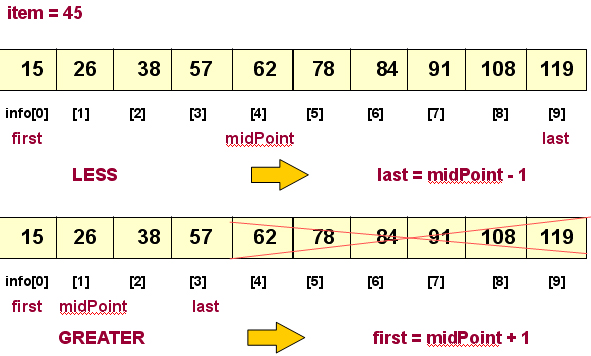
Second step
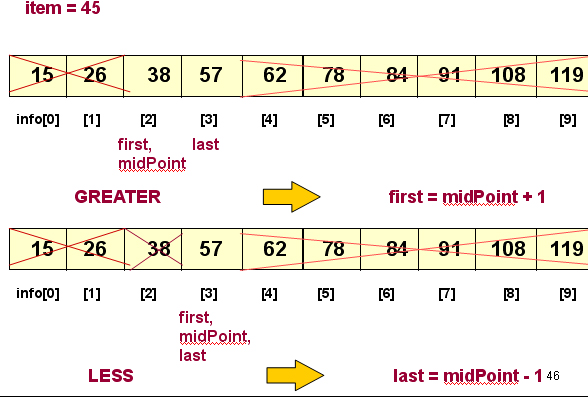
3rd Step
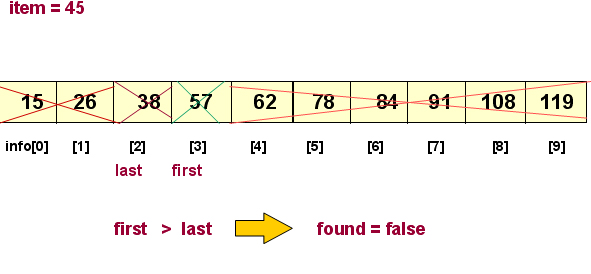
|