Project 2- Image Processing Application
Due start of class Oct. 26
DO NOT USE OpenCV processing methods EXCEPT for HoughLines & video capture and use of Mat class ---everything else you must write the code to do edge detection, thresholding, etc. This is the POINT of this project
eval guidelines
1) extend what you did in previous exercise in creating an OpenCV based Android application
2) learn how to process with your own algorihtm (not one provided by OpenCV) each pixel in the image and alter it.
-
reason: OpenCV provides some basic algorithms but, sometimes you need to create your own, or modify an alogirthm to your needs and the OpenCV code wont work
3) you learn how to go through the features detected by an OpenCV Hough Line Transform and make simple decisions on them to select the best lines
-
reason: OpenCV provides some basic algorithms but, sometimes you need to create your own, or modify an alogirthm to your needs and the OpenCV code wont work
Alter exercise A3 so that you add the following:
1) Spinner Option: "Edgy"
a) convert to greyscale using OpenCV method
b) take Laplacian of image and get something like this:
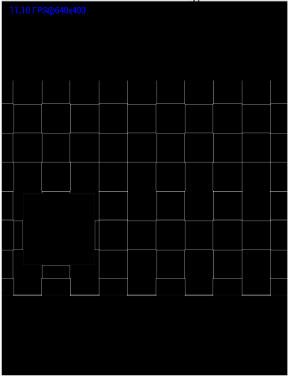
c) Threshold the
edge image into a binary_edge image and get something like this:
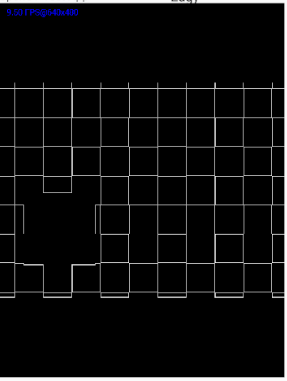
d)
Cycle through the image row by row and at each pixel set the final image equal to a bitwise AND between the original color image and a red Image (all pixels red) only where the binary_edge pixel value is =255 and you will get:
RED Image
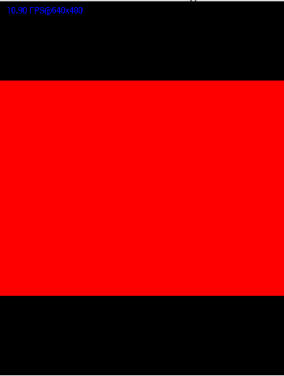
|
Final Image
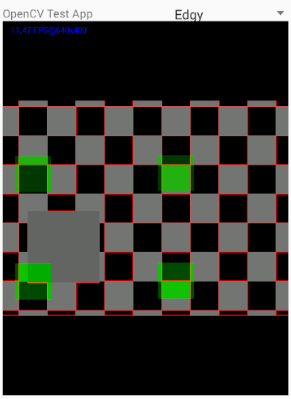
|
2) Spinner Option: "Best Lines"
a) convert to greyscale and perform Laplacian edge detector on live video images
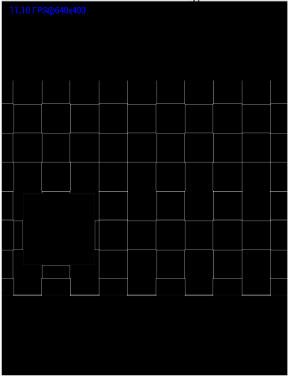
b)
perform Hough Line Transform ***using OpenCV*** (this is the only OPENCV processing method call you can use in this project) on Laplacian edge images and draw all the lines in blue on top of the original color image. Regarding the parameters for the HoughLines method:
-
You will choose the threshold value to be 1/4th the minimum of the width and height of the image.
-
You should choose the resolution for rho at 1 and for theta Math.PI/180.
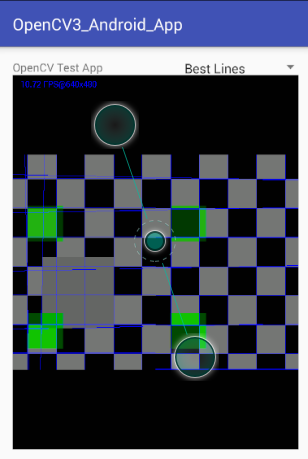
c) cycle through detected Hough Lines and choose lines that are within +/- 30 degrees of vertical (you will need to calculate the slope of each line) and in that set, choose the top 10 lines.
d) draw the lines selected in b in the color red on top of the image produced from step b
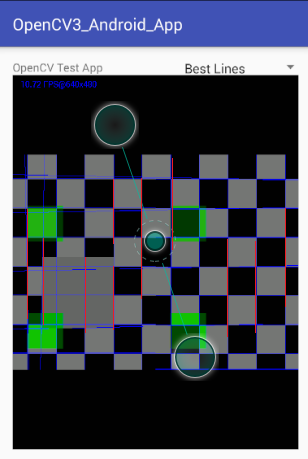
1) Word Document (click here for template you MUST use) that details the following:
- Section 1 Execution Instructions: Instructions for me to download and run your code. YOU NEED to show me screen shots of you doing this from your uploaded blackboard code.....this forces you to make sure that I can run your code. You MUST have the following screenshots AND give description on what to do:
- screenshot 1.1 = screen shot of your files uploaded to Project 2 turn in folder on blackboard
- screenshot 1.2 = directory view of "temp" directory you unzipped file to showing the unziped files and directory structures.
- screenshot 1.3 = Android Studio project settings - what version of Android SDK you are runing and version of OpenCV
- screenshot 1.4 = AVD you are using - show screen shot of setting AND description of hardware you are running
- Section 2 Code Descpription: A describing how code is structured and the state of how
it works.
Give a describption for each filename listed.
- Section 3 Testing: here you give screen shots of you running the various stages of the program as detailed here:
- section 3.1:app -running Edgy
- screenshot 3.1 a= first image from video showing results of edgy processing
- screenshot 3.1b= second image from video showing results of edgy processing
- section 3.2: app -running Best Lines
- screenshot 3.1 a= first image from video showing results of Best Lines processing
- screenshot 3.1b= second image from video showing results of Best Lines processing
- Section 4 Comments Optional any comments you have regarding your code (necessary if you code is not working, you need to tell me in detail what the problem is or what is missing)
2) URL of YouTube Video #1 using Mobizen app to record you using the app
3) URL of YouTube Video #2 which is a video containing:
a) demo of app
b) showing code you created and go over each algorithm for the two Edgy and Best Lines processing options
c) disucss anything not work and why, what did you implement
|