Image Indexing
How do we store images in memory? Well there are two basic ways, one is via a pointer to a 1-D array and there is also the possibility of having a multidimensional array. The possiblities are discussed below.
Pointer to 1D Array, Greyscale Image
Problem: Have an Image of any arbitrary size and want to store
it. You can use a defined 2-D array like
int Image[10][10];
But this only works if you have a 10x10 image.
Solution: You should use the concept of a pointer and
dynamically allocate memory to it. In this case, you will be representing
a 2D image by a 1D array.
First, Lets review the use of Pointers
in C/C++.
- Next we must allocate memory to our point to contain all of our
pixels:
Example: I =
malloc(Image_Width*Image_Height);
Note: sizeof(unsigned char) = 1
- Now we can fill our pointer I which can be treated like a 1D array. How can we do this?
Answer: Store 1 row of data after the other in the
same fashion as we have discussed visiting all the pixels in an image
which is called "row scanning".
If we had a 2D array I2[r][c]where r = row and c=column and we wanted to store its
pixels in our 1D array, this is the algorithm that would do it:
for(r=0; r<Image_Height;
r++)
for(c=0;
c<Image_Width; c++)
I[r*Image_Width + c]
= I2[r][c];
Looking in more
detail, this is how the pixels (r,c) are stored in the 1D array:
Where W = Image_Width
|
2D Array Greyscale Image
Another possibility is to create a multi-dimensional array I[r][c] dynamically. See See 3240 discussion of arrays.
Also, take a look at the following exercise that has some code for creating such arrays.. it is not complete...it is an exercise. |
Pointer to 1D Array , Color Image
Now consider a color image instead of a greyscale image.
- we must store for each pixel the red, green and blue values
- Hence now, instead of one grey value, for each pixel we store its red,
green , blue values in this order.
- Below is a partial program illustrating how this is done. Notice
for each pixel we store 3 values -> Hence the
multiplicative factor of 3 througout!
unsigned char *I;
I =
malloc(Image_Width*Image_Height*3);
/*to access Red value for Pixel
(r,c) */
red_at_r_c =
I[r*Image_Width*3 + c*3];
/*to access Green value for Pixel
(r,c) */
green_at_r_c =
I[r*Image_Width*3 + c*3 + 1];
/*to access Blue value for Pixel
(r,c) */
blue_at_r_c =
I[r*Image_Width*3 + c*3 + 2];
This can be viewed as follows:
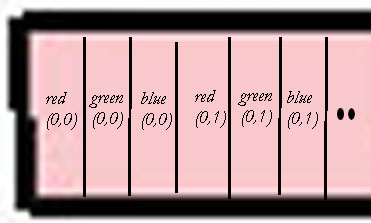
|
Multidimensional Array, Color Image
- Case 1: 2D array, I[row][column] where store a data stucture value "color" that contains red, green, blue fields
- Case 2: 3D array, I[row][column][x] where x indicates the color field, if x=0 means red, if x=1 means green and if x=2 means blue.
|
|