No web.xml and Annotations instead --- Servlets 3.*
- Since Java EE 6 and Servlet 3.0 you are not required to have a web.xml file but, can instead use annotations to
signify information you would have found in the web.xml deployment description
Here is an example of a directory structure using GlassFish (and this is in Eclipse IDE with GlassFish pluggin)
Notice how there is NO web.xml file in the WEB-INF directory, only the container specific sun-web.xml file is there.
Resources
Notice
- Most of the examples in our website will be devoid of annotations to make the code more readable ---however, you should be aware of these annotations --luckily using IDES like Elcipse will help you out and even autogenerate them for you.
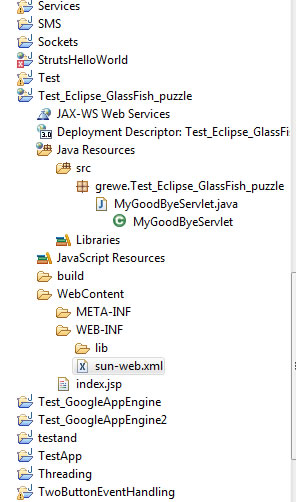
<<<as we will see this Servlet contains annotations that specify URL mapping
<<<container will deploy this using this information
<<<No web.xml file in the WEB-INF directory, only the sun-web.xml GlassFish specific descriptor file
- rightclick on ProjectName->JavaResources->src and select New->Servlet
NOTE: There is NO web.xml file in WEB-INF directory>>>>>
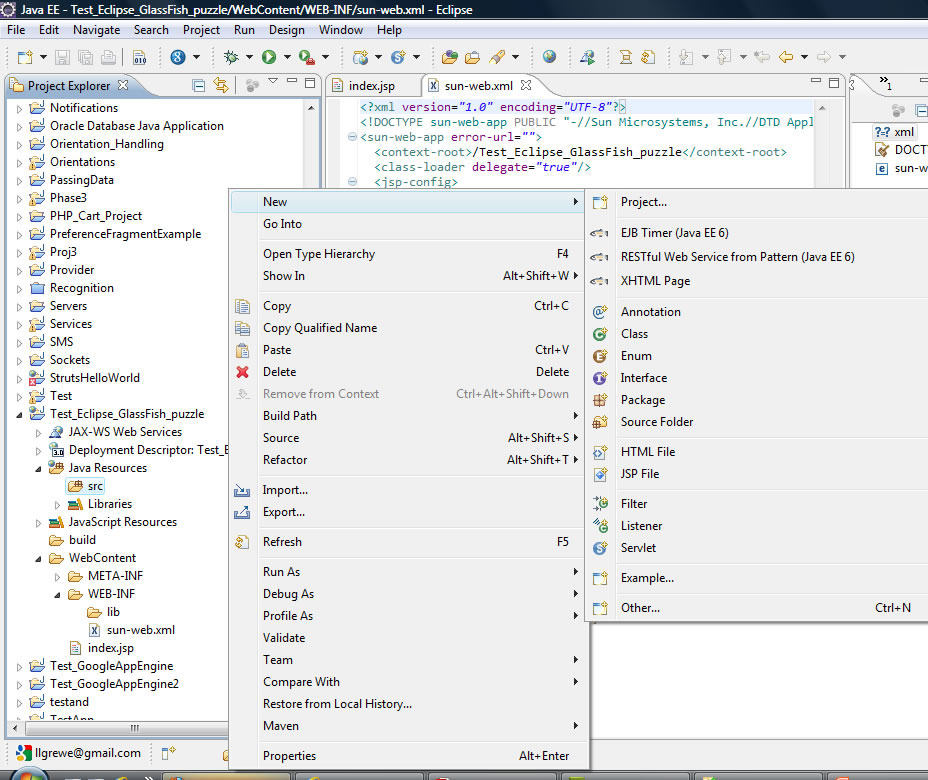
This will pop-up the following window, where you need to type in the package name, name of class, what kind of servlet you are extending
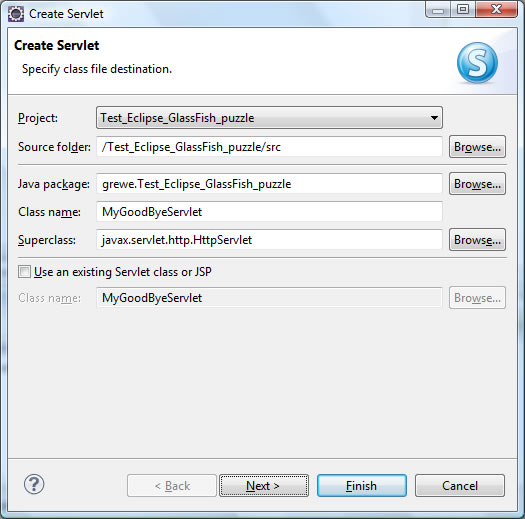
Hit Next if you wish to type in any intialization parameters OR change the URL mappings (these were things in old Servlet standard you put in web.xml---you still can)
Next select any methods you want to overwrite, and other class options
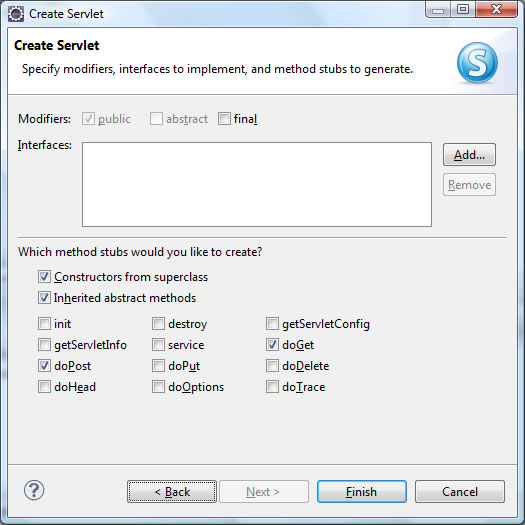
The following code will now be generated under you src folder
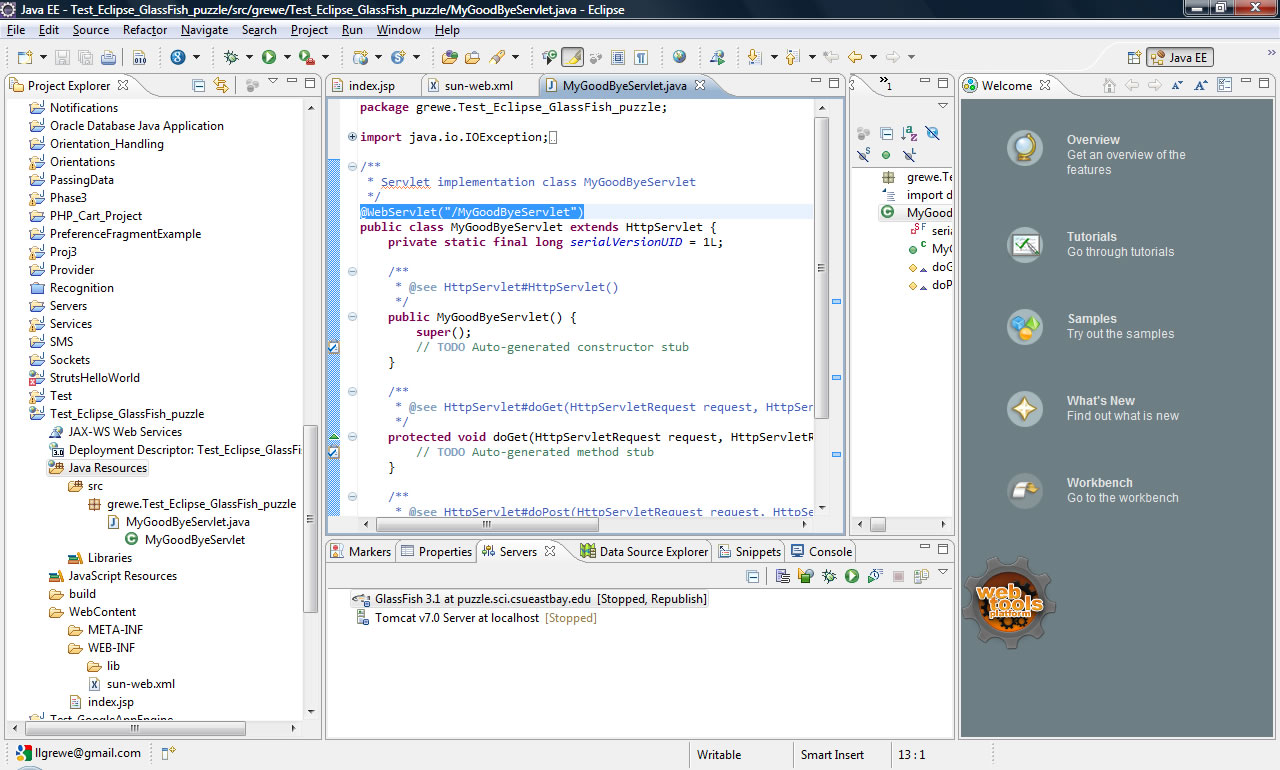
Lets look at the Code and explain it
package grewe.Test_Eclipse_GlassFish_puzzle;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class MyGoodByeServlet
*/
@WebServlet("/MyGoodByeServlet") // this @WebServlet annotation to give servlet mapping for this class
public class MyGoodByeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public MyGoodByeServlet() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
PrintWriter out = response.getWriter(); //I added some code to say goodbye out.println("Goodbye CS6320");
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
here it is running ....see the URL to invoke ends in the annotated URL mapping /MyGoodByeServlet
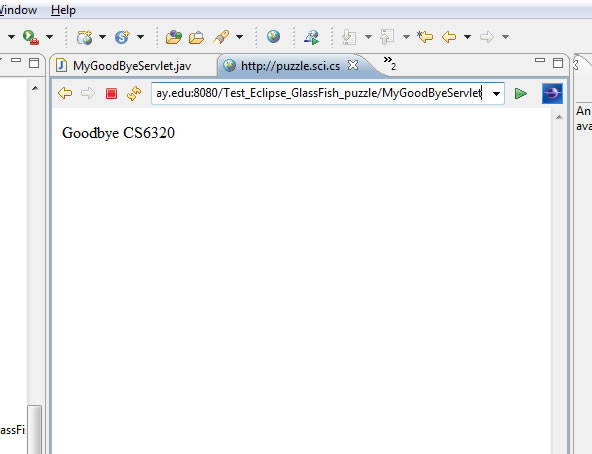
javax.servlet.annotation Types @XXXXXXX
annotations that allow users to use annotations to declare servlets, filters, listeners and specify the metadata for the declared component.
Annotation Types Summary |
HandlesTypes |
This annotation is used to declare the class types that a ServletContainerInitializer can handle. |
HttpConstraint |
This annotation is used within the ServletSecurity annotation to represent the security constraints to be applied to all HTTP protocol methods for which a corresponding HttpMethodConstraint element does NOT occur within the ServletSecurity annotation. |
HttpMethodConstraint |
This annotation is used within the ServletSecurity annotation to represent security constraints on specific HTTP protocol messages. |
MultipartConfig |
Annotation that may be specified on a Servlet class, indicating that instances of the Servlet expect requests that conform to the multipart/form-data MIME type. |
ServletSecurity |
This annotation is used on a Servlet implementation class to specify security constraints to be enforced by a Servlet container on HTTP protocol messages. |
WebFilter |
Annotation used to declare a servlet filter. |
WebInitParam |
This annotation is used on a Servlet or Filter implementation class to specify an initialization parameter. |
WebListener |
This annotation is used to declare a WebListener. |
WebServlet |
Annotation used to declare a servlet. |
@WebServlet annotation attributes
---only urlPatterns is required.
- name - Name of the servlet - optional
- asyncSupported=true/false - Specifies weather the servlet supports asynchronous processing or not.
- largeIcon - large icon for this Servlet, if present - optional
- smallIcon - small icon for this Servlet, if present - optional
- description - Servlet description - optional
- initParams - Array of @WebInitParam, used to pass servlet config parameters - optional
- loadOnStartup - Integer value - optional
- displayName -Servlet display name - optional
- urlPatterns - Array of url patterns for which the sevlet should be invoked - At least one url pattern is requried
Example:
@WebServlet(asyncSupported = false, name = "HelloAnnotationServlet", urlPatterns = {"/helloanno"}, initParams = {@WebInitParam(name="param1", value="value1"), @WebInitParam(name="param2", value="value2")} )
|