Accessing Databases using Java: JDBC
Now, that you know to get at the data you have to use SQL commands/querries,
you need to solve the problem of gaining access or in other words connecting
to the database so you can issue these SQL commands. For us
in Internet Programming, we will explore how we can do this with Java
and JDBC. There are other solutions, some companies like Oracle
will have their own special version of SQL (e.g. PL/SQL) and server software
that can be invoked to connect to the database and issue SQL commands.
Java and JDBC connecting to a Database
from
www.oracle.com
Features of Java-JDBC Database System Architecture
- Easily Change Database** you are using
if:
- It supports SQL (which most do).
- Has "Java Driver".
- You need Driver on each client machine.
**This
should be compared to using proprietary langauges provided by the Database
manufacturer which would require a major re-writting of the code used.
Driver Manager
loads Database drivers and manages connections between appliation
and driver.
Driver
translates API calls into database specific calls.
Connection
session between application and database
Statement
SQL statement
Metadata
info about returned data, database and driver.
ResultSet
retured data from an SQL querry....returned in form of a
table (rows and columns)
|
STEPS in Java Code to Connect
(regardless of driver choice)
The Process (see below for example)
- Import classes
- Use DriverManager to load the JDBC driver
- Class.forName("X.jdbc.odbc.Z");
- X.jdbc.odbc.Z is a class that must be on your system and registered
by your Operating System's ODBC manager
- Create Connection object to specific database
- String s = "jdbc:odbc:dbname"
- Connection c = DriverManager.getConnection(s)
- Create Statement object to make SQL calls
- Execute query using Statement from 4.
- ResultSet r = statement.executeQuery("SELECT * FROM table0");
//just an example
- Results are returned in a ResultSet object (e.g. r in 5)
- Process results as desired
- Repeat steps 4-7
- Close ResultSet
- Close Statement
- Close Connection
|
EXAMPLE for ORACLE
- You will use OCI for Java Applications
- You will use Thin for Java Applets or Applications.
What you need to do:
1) Have your database administrator load the Java JDBC driver for
the database you are using.(you will use OCI for a Java Application
and Thin for an Applet...see above)
2) You with your DBA (database administrator) set up your
database table(s) and enter in the data as desired (note you can
also if you wish to have data entry take place through an interface
to your Java program).
3) Now write the code. Steps in the code (SEE
EXAMPLE):
1)Import java.sql.*;
2)Load JDBC Driver using Class.forName() method.
3)Connect to Driver using DriverManager.getConnection(...);
If one of the drivers you loaded in step 2 recognizes the
JDBC URL supplied to the method DriverManager.getConnection
, that driver will establish a connection to the DBMS specified
in the JDBC URL. The DriverManager class, true to its name,
manages all of the details of establishing the connection
for you behind the scenes. Unless you are writing a driver,
you will probably never use any of the methods in the interface
Driver , and the only DriverManager method you really need
to know is DriverManager.getConnection .
4)Create an instance of the Statement class using
connection's createStatement()
method
5) Using this instance of Statement, execute various SQL
querries using executeQuerry(.) method.
Results are returned as a table, which is stored in an instance
of the class ResultSet.
6) Process the information in the instance of ResultSet as desired.
See methods such as next(), getString(.)
Examples Application Connection
Examples Applet Connection
Example Servlet Connection
IMPORTANT: you must add the get the appropriate Oracle jdbc jar file for the version of oracle from Oracle.com and upload the jar file to your WEB-INF/lib and configure your project properties to include it as a jar file under libraries in Eclipse (for Oracle 11.0.2.* ojdbc6.jar)
|
Running Compiled Example Above:
|
Specifying Database
you wish to connect to via JDBC
Uses Internet-standard URLs to identify database connections.
URL Specification will be given to you in documentation or examples
provided by the JDBC driver provider.
Below are examples for Oracle that we will use.
OPTION 1:
DriverManager.getConnection ("jdbc:oracle:oci8:@labdb", "userid",
"password");
-
URL = jdbc:oracle:oci8:@labdb
labdb represents the identity
of the DBMS stored in a client-side configuration file.
this can only be used on Applications
that have client-side configuration files setup
|
OPTION 2:
DriverManager.getConnection ("jdbc:oracle:oci8:@host :
port : sid", "userid", "password");
-
URL = jdbc:oracle:thin:@host:port:sid
host:port:sid represents the
identity of the DBMS.
should be used for Applications
or Applets using Thin Driver where do not wish to have client-side
configuration files setup.
|
-
-
ALTERNATIVES FOR CONNECTING
Connecting Directly from Applet to DB through Oracle's Net8
Connection Manager
from www.oracle.com
- Gets around SECURITY problem
that Web-Server and Database Server Must be the same machine.
- Oracle
Connection Manager is a multipurpose networking
service for Oracle environments. Included with Oracle8 Enterprise
Edition, Oracle Connection Manager provides client connection
concentration, client connection access control, and multiprotocol
connectivity.
- Using the Connection Manager requires two steps:
- Installing and running the connection manager on the
Web Server host.
- Using a connect string in the applet that routes through
the Connection Manager.
- Using the proper connect string is critical to
access a remote database through Connection Manager. The
following connect string will connect a JDBC applet to a
database on host DB_server, at port 1521 and
SID ORCL:
- Connection conn = DriverManager.getConnection
("jdbc:oracle:thin:" + "@(description=(address_list="
+ " (address=(protocol=tcp)(host=CM_server)(port=1610))"
+ " (address=(protocol=tcp)(host=DB_server)(port=1521)))"
+ " (source_route=yes)" + " (connect_data=(sid=orcl)))",
"scott", "tiger");
- The first element in the "address_list" entry
represents the connection to the Connection Manager.
- The second element represents the destination
database. The "source_route" entry tells JDBC that
it should pass the second address to the Connection
Manager. Note that if the source_route parameter
is omitted, JDBC will treat the second address as
a fall back address in case the first one does not
respond.
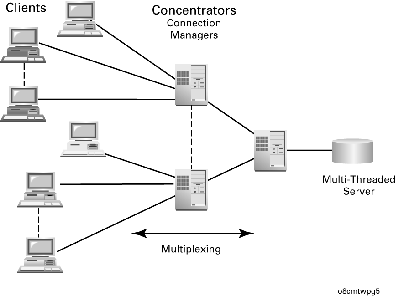
GOOD THING: One Oracle
Connection Manager connection represents only one socket used,
even if the Connection Manager is serving 100 clients. So,
when multiple Oracle Connection Managers are employed, thereby
connecting multiple clients, the total number of clients connecting
to a single database grows exponentially. The benefits of
using Oracle Connection Manager include support for large
client populations and the ability to identify and monitor
the actual users connected. Additionally Oracle Connection
Manager is an ideal implementation for very many "heavy" or
continuous client applications.
|
|
|