Deadlock
- when synchronizing access to data or methods, be careful, you can
end up in deadlock
- occurs when there is a cycle of threads waiting for each other.
Example: Suppose we have the following code, and gerenerate
2 threads each waiting on data from the other one:
class Foo{
private int x=0;
synchronized void setMaximum(int v, Foo f) { x = Math.max(v,f.getValue());
}
synchronized int getValue(){ return x; }
}
Now lets use the class as follows:
Foo a = new Foo();
Foo b = new Foo();
a.setMaximum(2,b);
b.setMaximum(3,a);
This can cause the following execution:
Thread 1 |
Thread 2 |
aquire lock on a |
|
|
aquire a lock on b |
attempt call to b.getValue() |
|
wait* |
|
|
attempt call to a.getValue() |
|
wait* |
*wait was invoked by the JVM switching which
is the current thread of a set of equal priority threads.
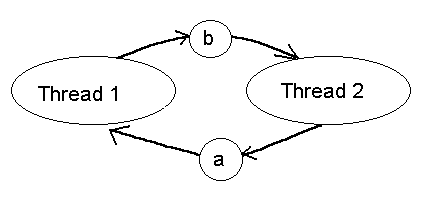
|
Avoiding Deadlock
You can avoid problems like the example above by making sure
that synchronized methods never call (directly or indirectly) synchronized
methods on other objects (that could be locked by other threads).
|
|