Overview of OOP
OOP = Object Oriented Programming.
Objectives:
Class = a template representing a self-contained element
of a computer program
(i.e. a module) that represents related information and has
the cability to accomplish specific tasks through the invokation
of methods.
Object = a particular instance of a class.
Instance = same thing as Object.
An OO Program = A set of classes, pluseobjects created from those
classes and used as needed.
class library = group of classes that you can use in your
own program. In Java, we also call this a package (a group of
related classes).
- Packages in java are called java.*
- by default, your java classes have access only to the pacakage
java.lang You must refer to other classes explicitly (
e.g. java.awt.Color) or import the package and then just use
the classname.
Example
Dog Class and several Dog objects/instances
Inheritance
- a mechanism that enables one class to inherit all the
behaviors and attributes of another class.
subclass = a class that inherits from another class.
superclass = a class that is its inheritance to another class.
Example
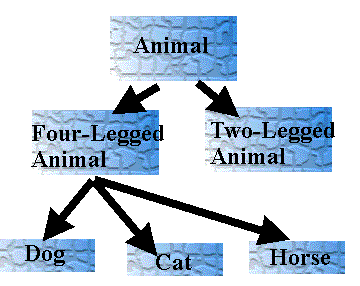
Hierarchy of Animal Classes.
Note: Subclasses can override methods they have inherited
if they want to change the corresponding behavior. For example,
the method to calculate the Expected Lifespan for each Class
of Dog, Cat, and Horse may be different from thier superclass
Four-Legged Animal.
Interfaces
- is a collection of methods that indicate a class has some
behavior in addition to what it inherits from its superclasses
- It is the way that a set of method names, without definitions.
By this I mean the method's
interface is presented but not implemented. This will allow similar behavior to be
duplicated acrros different parts of the class hierarchy which is achievable in C++ through
multiple inheritance which Java does not allow.
- Can not declare an object of this class....must create a new class that extends this and
implements all of the unimplemented methods.