Dependency Injection
-
a technique for assembling applications from a set of concrete classes that implement generic interfaces
-
create loosely coupled systems as the code you write only ever references the generic interfaces that hide the concrete classes
Dependency Injection origin in a famous blog post by Martin Fowler
see http://martinfowler.com/articles/injection.html
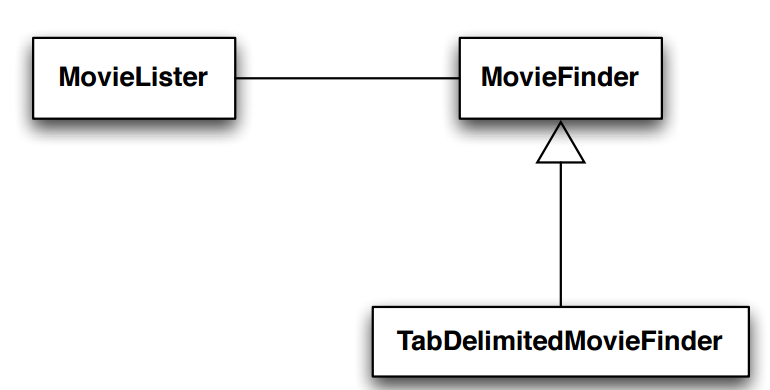
A MovieLister class = lists movies with certain characteristics after being provided a database of movies by an instance of MovieFinder.
MovieFinder = interface
TabDelimitedMovieFinder = is a concrete class that can read in a movie database that is stored in a tab-delimited text file
Goal: Loosely coupled system = to avoid having our code depend on concrete classes
DO NOT want depencencies on concrete code or hard coded values.
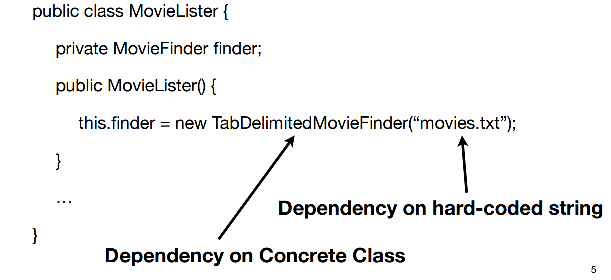
Problems with this code:
-
a reference to a concrete class that implements MovieFinder
-
a reference to a hard-coded string
-
The name of the movie database cannot change without causing MovieLister to be changed and recompiled
-
The format of the database cannot change without causing MovieLister to be changed to reference the name of the new concrete MovieFinder implementation
Solution:
public class MovieLister {
private MovieFinder finder;
public MovieLister(MoveFinder finder) {
this.finder = finder;
}
…
}
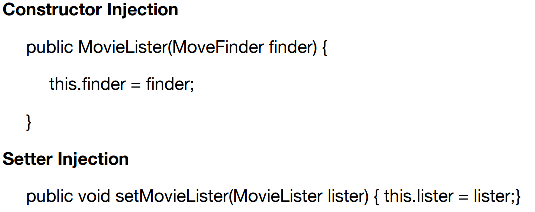
The IDEA
classes in an application indicate their dependencies in ABSTRACT ways
-
MovieLister NEEDS-A MovieFinder
-
Main NEEDS-A MovieLister
-
A third party injects (or inserts) a class that will meet that dependency at run-time --> The “third party” is known as a “Inversion of Control container” or a “dependency injection framework”
The CONCEPT -- from a non-framework perspective "Dependency injection is basically providing the objects that an object needs (its dependencies) instead of having it construct them itself"
The CONCEPT - from a more framework perspective "dependency injection is a software design pattern that implements inversion of control for resolving dependencies. A dependency is an object that can be used (a service). An injection is the passing of a dependency to a dependentobject (a client) that would use it."
There are many frameworks that implement the Dependency Injection Framework.
-
Spring, JSF for Server side Java
-
How many current Dependency Injection Frameworks work = classes, interfaces + meta data files (often xml) + Inversion of Control Container
{ a set of components (concrete classes + interfaces) } + {a set of configuration metadata} + Dependency Injection Framework --> small set of code that gets acces to the "Inversion of Control Container" that retrieves first object from that container representing the needed interface.
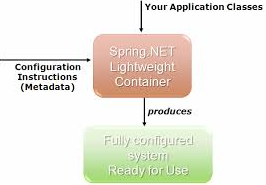 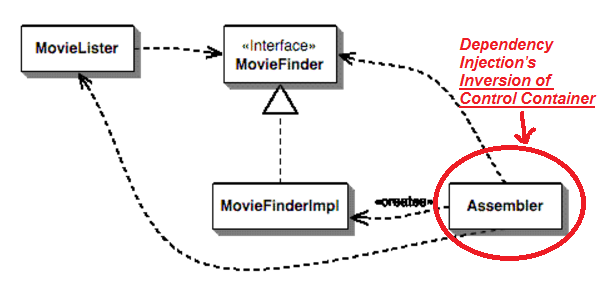
|