Class from Oracle that uses Swing to create a GUI program to convert Clesius to Farenheit
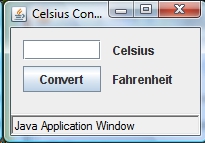
Click Here for local copy of Oracle provided instructions on how this was created using Netbeans interface. The GUI creation was done with
public class CelsiusConverterGUI extends javax.swing.JFrame {
/** Creates new form CelsiusConverterGUI */
public CelsiusConverterGUI() { initComponents(); }
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor. */
// <editor-fold defaultstate="collapsed" desc=" Generated Code ">//GEN-BEGIN:initComponents private void initComponents() {
//create GUI components (defined at bottom of classs) for this ClesiusConverterGIU class
tempTextField = new javax.swing.JTextField();
celsiusLabel = new javax.swing.JLabel();
convertButton = new javax.swing.JButton();
fahrenheitLabel = new javax.swing.JLabel();
//set up some attributed of this JFrame class
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Celsius Converter"); celsiusLabel.setText("Celsius");
//setup properties of convertButton and Action Listener.
convertButton.setText("Convert");
convertButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
convertButtonActionPerformed(evt); }
});
//setup label
fahrenheitLabel.setText("Fahrenheit");
//set the Layout manger for this JFrame to be GroupLayout. and ADD GUI components to it
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(tempTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(celsiusLabel))
.addGroup(layout.createSequentialGroup()
.addComponent(convertButton)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(fahrenheitLabel)))
.addContainerGap(27, Short.MAX_VALUE))
);
layout.linkSize(javax.swing.SwingConstants.HORIZONTAL, new java.awt.Component[] {convertButton, tempTextField});
layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup()
.addContainerGap() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(tempTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(celsiusLabel))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(convertButton)
.addComponent(fahrenheitLabel))
.addContainerGap(21, Short.MAX_VALUE))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private void convertButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_convertButtonActionPerformed
//Parse degrees Celsius as a double and convert to Fahrenheit
int tempFahr = (int)((Double.parseDouble(tempTextField.getText())) * 1.8 + 32);
fahrenheitLabel.setText(tempFahr + " Fahrenheit");
}//GEN-LAST:event_convertButtonActionPerformed
/** * @param args the command line arguments */
public static void main(String args[]) {
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new CelsiusConverterGUI().setVisible(true);
}
});
}
//CLASS Variables, placed on Bottom by Java IDE that was used.
//
define various GUI components will add to our CelsiusConvertGIU.
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel celsiusLabel;
private javax.swing.JButton convertButton;
private javax.swing.JLabel fahrenheitLabel;
private javax.swing.JTextField tempTextField;
// End of variables declaration//GEN-END:variables
}
|