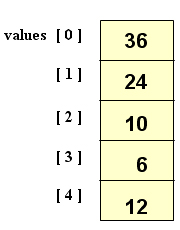
Compares neighboring pairs of array elements, starting with
the last array element, and swaps neighbors whenever they are not
in correct order.
On each pass, this causes the smallest element to “bubble up”
to its correct place in the array.
Snapshot of BubbleSort
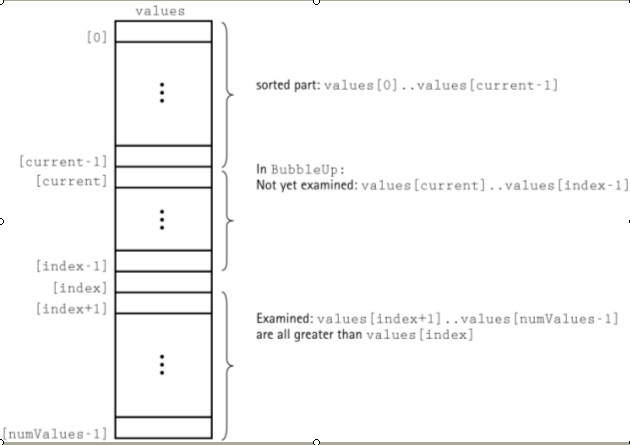
|
// CODE
template
void BubbleSort(ItemType values[],
int numValues)
{
int current = 0;
while (current < numValues - 1)
{
BubbleUp(values, current, numValues-1);
current++;
}
}
template
void BubbleUp(ItemType values[],
int startIndex, int endIndex)
// Post: Adjacent pairs that are out of
// order have been switched between
// values[startIndex]..values[endIndex]
// beginning at values[endIndex].
{
for (int index = endIndex;
index > startIndex; index--)
if (values[index] < values[index-1])
Swap(values[index], values[index-1]);
}
|