Implementation using Heap
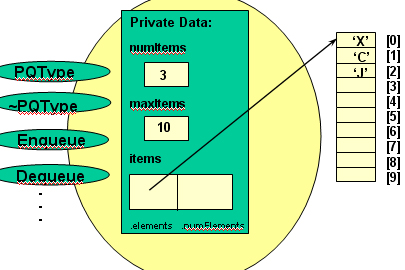
Dequeue = to read root value (highest priority) and remove
it
Set item to root element from queue
Move last leaf element into root position
Decrement length
Heapify
Enqueue = to insert new element in queue
It has to place the new element where it belongs in the queue.
Here we just stick it at the very end of the heap in the array
(increasing the size of the heap) and then move it up the chain
of parents until it is smaller than it's parent (if it is larger
than its parent then it doesn't hurt to swap it with it's parent,
the heap at that node will still satisfy the heap condition).
//PSEUDO-CODE
class FullPQ(){};
class EmptyPQ(){};
class PQType
{
public:
PQType(int);
~PQType();
void MakeEmpty();
bool IsEmpty() const;
bool IsFull() const;
void Enqueue(ItemType newItem);
void Dequeue(ItemType& item);
private:
Heap heap;
};
PQType::PQType(int max)
{
maxItems = max;
heap = new Heap();
heap.heapsize = 0;
}
void PQType::MakeEmpty()
{
heap.heapsize = 0;
}
PQType::~PQType()
{
delete [] heap.elements;
}
float PQType::Dequeue() {
if (heap.ISEmpty())
throw(EMPTYHEAP);
max = heap.getRoot(); //first element, root
//put last element at root
heap.setRoot(heap.elements[heapsize]);
heap.heapsize -=1;
Heapify(heap.elements,1);
return max;
}
float PQType::Enqueue(float new_value)
heap.heapsize = heap.heapsize + 1;
index = A.heapsize;
while (index > 1 && heap.getParent(index) < new_value )
{heap.elements[index] = heap.getParent(index);
index = parent(index); //get parent's index
}
heap.elements[index] = new_value;
}
|