Memory Allocation for Data
When a variable is declared, enough memory to hold a value of
that type is allocated for it at an unused memory location. This
is the address of the variable.
For example:
int x; //will take 2 bytes
float number; //will take 4 bytes
char ch; //will take 1 byte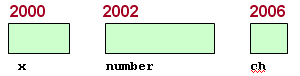
|
Kinds of Memory Allocation
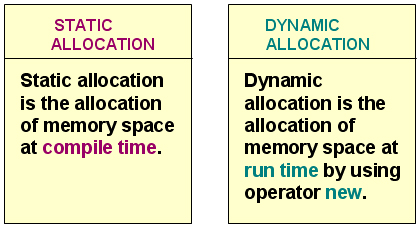
|
3 Kinds of Data in a Program
STATIC DATA: memory allocation
exists throughout execution of program.
static
long SeedValue;
AUTOMATIC DATA: automatically
created at function entry, resides in activation frame of the
function, and is destroyed when returning from function.
DYNAMIC DATA: explicitly
allocated and deallocated during program execution by C++ instructions
written by programmer using unary operators new
and delete
|
Using new operator (dynamic data allocation)
If memory is available in an area called the free store (or heap),
operator new allocates the requested object or array, and
returns a pointer to (address of ) the memory allocated.
Otherwise, the null pointer is returned.
The dynamically allocated object exists until the delete
operator destroys it.
Next
|
Using delete operator (dynamic data de-allocation)
The object or array currently pointed to by the pointer is deallocated,
and the pointer is considered unassigned. The memory is returned
to the free store.
|
Another example - Dynamic Array Allocation
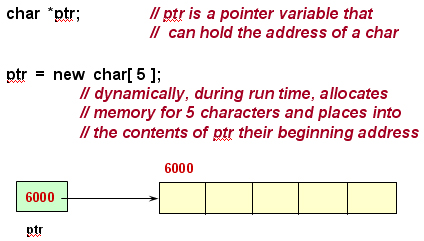
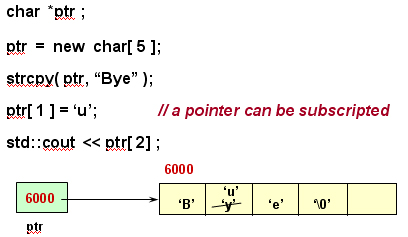
|
Reallocation
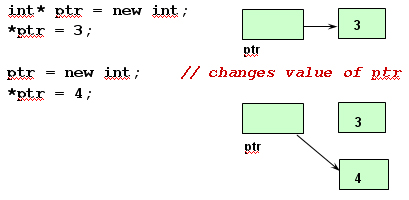
|
Memory Leak
A memory leak occurs when dynamic memory (that was created
using operator new) has been left without a pointer to it by the
programmer, and so is inaccessible.
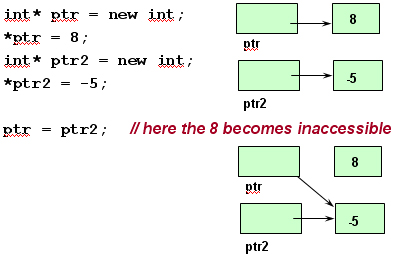
|