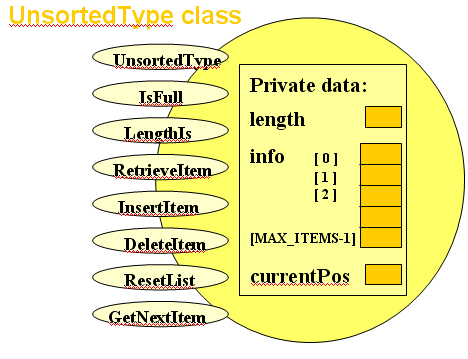
// SPECIFICATION FILE ( unsorted.h )
#include “ItemType.h”
class UnsortedType // declares a class data type
{
public : // 8 public member functions
void UnsortedType ( ) ;
bool IsFull ( ) const ;
int LengthIs ( ) const ; // returns length of list
void RetrieveItem ( ItemType& item, bool& found ) ;
void InsertItem ( ItemType item ) ;
void DeleteItem ( ItemType item ) ;
void ResetList ( );
void GetNextItem ( ItemType& item ) ;
private : // 3 private data members
int length ;
ItemType info[MAX_ITEMS] ;
int currentPos ;
} ;
// IMPLEMENTATION FILE ARRAY-BASED LIST ( unsorted.cpp )
#include “itemtype.h”
void UnsortedType::UnsortedType ( )
// Pre: None.
// Post: List is empty.
{
length = 0 ;
}
void UnsortedType::InsertItem ( ItemType item )
// Pre: List has been initialized. List is not full.
// item is not in list.
// Post: item is in the list.
{
info[length] = item ;
length++ ;
}
Before HSing inserted into list 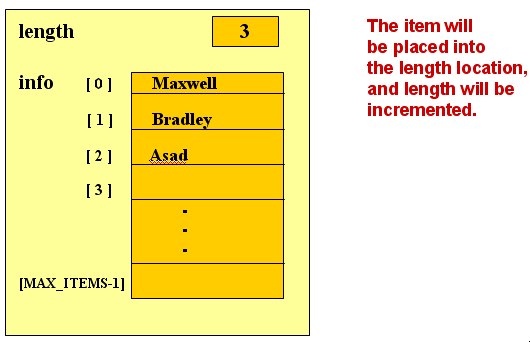
After HSing inserted into list
int UnsortedType::LengthIs ( ) const
// Pre: List has been inititalized.
// Post: Function value == ( number of elements in
// list ).
{
return length ;
}
bool UnsortedType::IsFull ( ) const
// Pre: List has been initialized.
// Post: Function value == ( list is full ).
{
return ( length == MAX_ITEMS ) ;
}
void UnsortedType::RetrieveItem ( ItemType& item, bool& found )
// Pre: Key member of item is initialized.
// Post: If found, item’s key matches an element’s key in the list
// and a copy of that element has been stored in item;
// otherwise, item is unchanged.
{ bool moreToSearch ;
int location = 0 ;
found = false ;
moreToSearch = ( location < length ) ;
while ( moreToSearch && !found )
{ switch ( item.ComparedTo( info[location] ) )
{ case LESS :
case GREATER : location++ ;
moreToSearch = ( location < length ) ;
case EQUAL : found = true ;
item = info[ location ] ;
break ;
}
}
}
Attemp to Retrieve "Ivan" from List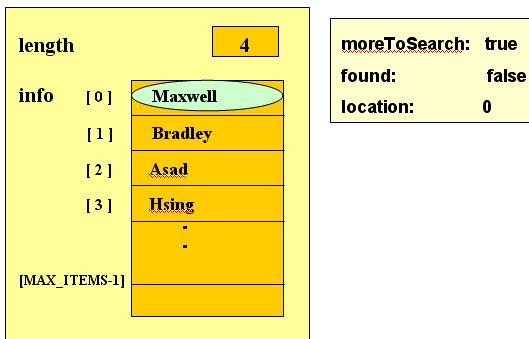
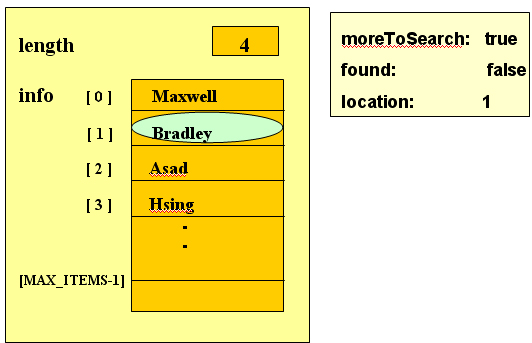
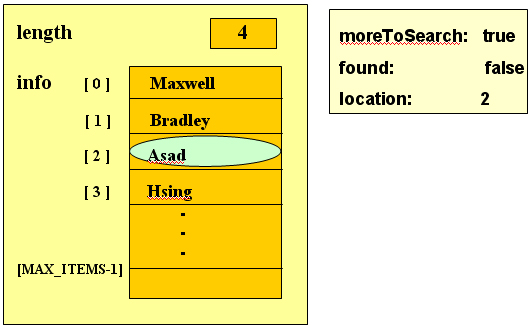
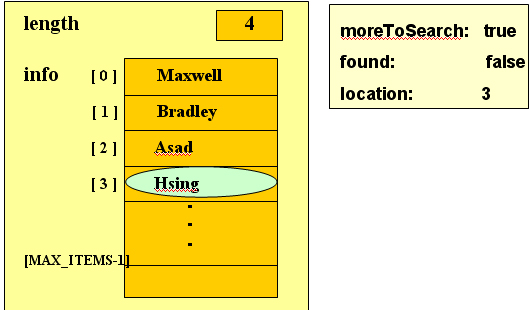
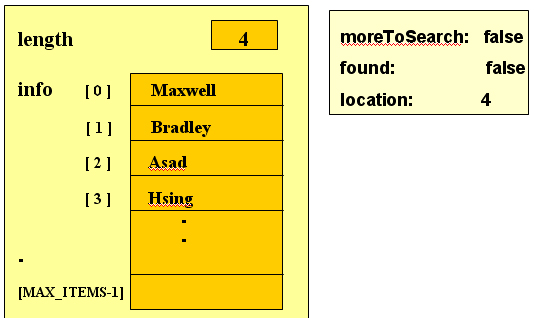
void UnsortedType::DeleteItem ( ItemType item )
// Pre: item’s key has been inititalized.
// An element in the list has a key that matches item’s.
// Post: No element in the list has a key that matches item’s.
{
int location = 0 ;
while (item.ComparedTo (info [location] ) != EQUAL )
location++;
// move last element into position where item was located
info [location] = info [length - 1 ] ;
length-- ;
}
Deleting Bradely from the List
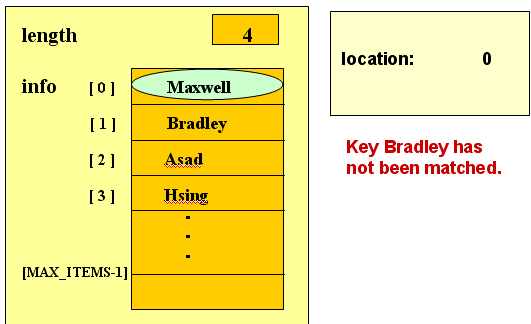
void UnsortedType::ResetList ( )
// Pre: List has been inititalized.
// Post: Current position is prior to first element in list.
{
currentPos = -1 ;
}
void UnsortedType::GetNextItem ( ItemType& item )
// Pre: List has been initialized. Current position is defined.
// Element at current position is not last in list.
// Post: Current position is updated to next position.
// item is a copy of element at current position.
{
currentPos++ ;
item = info [currentPos] ;
}
|