Breadth First Search - does a path exist from
vertex A to vertex B.
Here we figure out if a path exists from A to B by searching
from A in a breadth-first manner. If A and B are the same, the
search ends. Else we examine all of the vertices comming directly
out of A to see if they are B before going to a deeper level.
In our flight example, we first check for "non-stop"
flights before goint to 1-stop and then 2-stop and so on.
Depth versus Breadth first:
- depth first we back up as little as possible. Use a stack
- Last In, First Out (LIFO)
- breadth-frist we want to backup as far as possible to find
a route originating from the earliest vertices. Here we want
First In, First Out (FIFO)....so use a FIFO queue as our data
structure during search!!!!!
Example: Does a flight path exist from Austin to Washington?
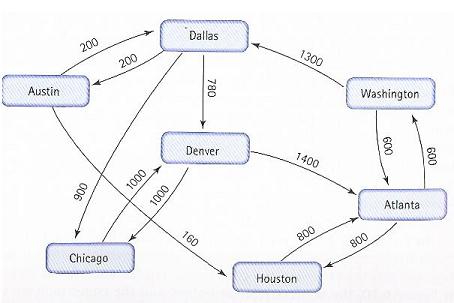
Intial PsuedoCode:
BreadthFirstSearch(A, B)
{
Set found to be false
for All Vertices
v comming out of A
queue.Enqueue(v)
//put on queue all vertices comming out of A 1-stop
do{
queue.Dequeue(vertex)
//pop of vertex on top of stack.
if
vertex = B //if
you are at the ending vertex you are done
Write
final vertex
Set
found to be true
else
Write
this vertex
Enqueue
all adjacent vertices onto queue
} while !queue.IsEmpty() AND !found
if(!found) //exhuasted
all paths and could not find path to B
Write "Path
does not exist"
}
Problem with above code:
- can cycle and revisit a vertex more than once
- could get into an infinite loop
Solution:
- mark vertices we have already visited and do not put them
on the path a second time.
- 3 new operators:
MarkVertex(VertexType vertex)
IsMarked(VertexType vertex)
- returns true/false if vertex marked or not.
ClearMarks() - sets marks
for all vertices to false.
code
Other Issues:
- What is printed out in the code presented here is the result
of the search....even the dead-ends that were found before backing
up to find the correct path. Consider the question: is there
a path from Auston to Washington. This is what are program would
print out: Auston -> Dallas -> Houston -> Chicago ->
Denver. -> Atlanta -> Washingto But,
we dont really want to visit Houston or Chicago!!!!
- This code does not necessarily find the "BEST" path.
See shortest path problem.