Arrays
Logical Level
- consists of finite, fixed-size collection of ordered hmogenous
(same type) elements
- component selection = direct access (do not have to first
access preceeding elements).
|
Application Level
Storage for lists of Things.
e.g. Name List, Customer List, Products list
|
Implementation Level
type Array[num];
- indexed from 0 to num-1
- Number of elements num
- Base Addres = ? (run time variation)
- Size of Element = sizeof type in bytes
float values[5];
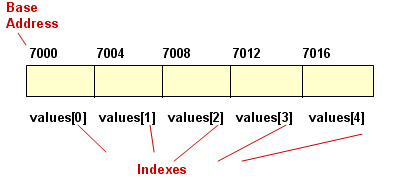
|
Q: Arrays in C++ are always passed by reference (e.g. type function(int
array[],...) )
How can we prevent change to an array?
A: Declare array as a const parameter
type function ( const int array[], .....)
now can only access elements or array but not alter their contents.
|
N-D arrays
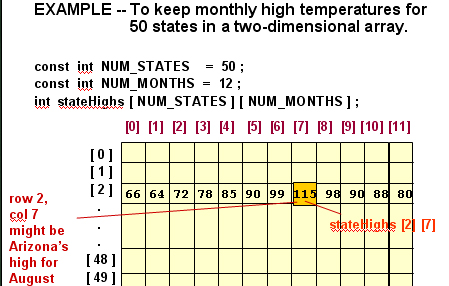
- In memory, C++ stores arrays in row order. The first row
is followed by the second row, etc.
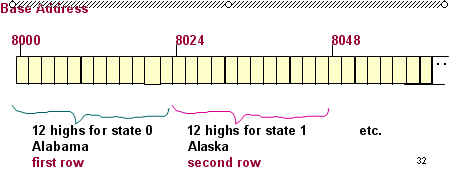
|
Arrays cannot be assigned one to another, and cannot be the return
type of a function.
|
Using typedef with arrays
helps eliminate the chances of size mismatches between formal
and actual parameters
typedef int StateHighsType [ NUM_STATES ] [ NUM_MONTHS
] ;
typedef int StateAveragesType [ NUM_STATES ] ;
void findAverages( const StateHighsType stateHighs , StateAveragesType
stateAverages )
{ . . . } |
const int NUM_DEPTS = 5;
// mens, womens, childrens, electronics, linens
const int NUM_MONTHS = 12 ;
const int NUM_STORES = 3 ; // White Marsh, Owings Mills,
Towson typedef long MonthlySalesType [NUM_DEPTS] [NUM_MONTHS]
[NUM_STORES];
MonthlySalesType monthlySales; |
|
Deleting an Array
To delete an array, we need to tell the compiler we are deleting
an array, and not just an element. The syntax is:
delete [] arrayName;
Deleting 2d arrays takes a little more work. Since a 2d array is
an array of arrays, we must first delete all the elements in each
of the arrays, and then we can delete the array that contains them.
Assuming we had created a 2d array called 2dArray, we could delete
it as follows: for(int i=0; i < (number of arrays); i++)
delete
[] 2dArray[i];
delete [] 2dArray;
|
|